It’s been a while that I’ve been setting up a private computer. For a long time I only had my old laptop, where I had not much motivation to tinker and optimize. Now that I have my new desktop computer (yay!), I’ve spent some time arranging the workspace / desktop. And of course I’m using KDE, as I have been for so many years.
The Plasma 5 system monitor is pretty cool, because it’s very configurable, and each of the graphs can be placed as Plasma widget on the desktop or in any panel. So I set up some nice graphs for SSD I/O, network throughput, CPU, memory, temperatures etc.. But then I thought: Can’t I also get some info from my Internet router, e.g. the current download rate of the whole house (as this PC is by far not the only device using the Internet connection). Or when it last reconnected, and what the external IP address is.
So I looked a bit into the TR-064 protocol and found a really nice Python module – fritzconnection by Klaus Bremer – to interface with my router (an AVM FRITZ!Box 5530 Fiber). It should also work with pretty much any other FRITZ!Box model, which is a very common Internet router throughout Germany. You can simply get it by running pip install fritzconnection
.
So after a few minutes I was able to query the desired data from the router with very few lines of code, like so:
os.environ['FRITZ_USERNAME'] = 'user' os.environ['FRITZ_PASSWORD'] = 'password' fc = FritzStatus(address='fritz.box') print('WAN Download: ' + fc.str_transmission_rate[1])
I found that querying it more than once every 5 seconds doesn’t make sense, because it doesn’t update the numbers more often than that. So a loop with a 5 second sleep it is.
But I also wanted to get the data into my nice panel, together with the CPU load etc… And by chance I stumbled over the Do It Yourself Bar – funny name, but it kind of fits: It’s a bar-shaped Plasma widget that you can populate via DBUS messages through any kind of script – typically Shell scripts but of course Python scripts work just as well.
So I modified my “text-only” Python script to send DBUS messages and hooked it into the DIY bar – and suddenly I have the data queried from the router in my KDE panel, with configurable colors and all!
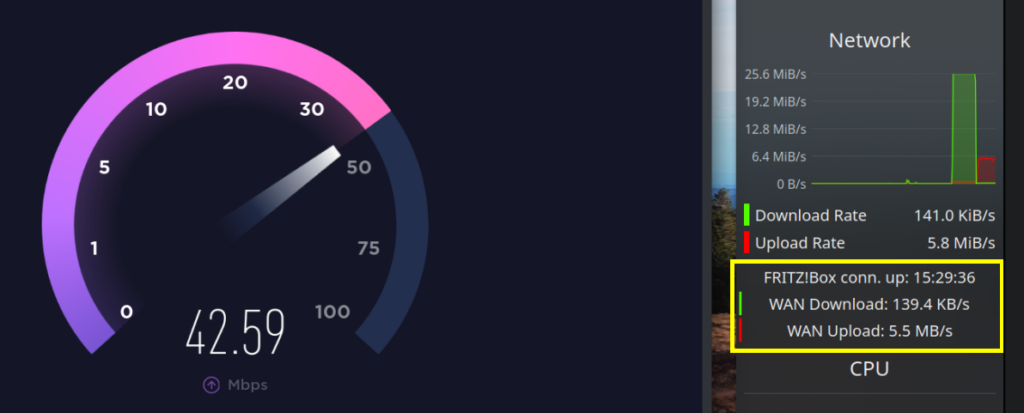
Hovering over the “FRITZ!Box conn. up: …” label also shows the external IP address, and clicking on it would open the browser with the FRITZ!Box’s web interface.
Here is the current Python script and my Pull Request.
#!/usr/bin/python # Prerequisites: # - Have a FRITZ!Box Internet router that is reachable at the address http://fritz.box # - Add a user + password to your FRITZ!Box (via the web interface) # - Run `pip install fritzconnection` to get the handy Python module by Klaus Bremer # (see https://fritzconnection.readthedocs.io) import os, sys, time from fritzconnection.lib.fritzstatus import FritzStatus os.environ['FRITZ_USERNAME'] = 'scriptuser' os.environ['FRITZ_PASSWORD'] = 'the_password' fc = None while not fc: try: fc = FritzStatus(address='fritz.box') except: data = '| A | FRITZ!Box not reachable | Have you changed username and password in get-fritz-wan-stats.py? Is your network up? | |' os.system('/usr/bin/qdbus org.kde.plasma.doityourselfbar /id_' + sys.argv[1] + ' org.kde.plasma.doityourselfbar.pass \'' + data + '\'') pass time.sleep(5) while fc: data = '| A | FRITZ!Box conn. up: ' + fc.str_uptime + ' | External IP: ' + fc.external_ip + ' | xdg-open http://fritz.box |' data += '| B | WAN Download: ' + fc.str_transmission_rate[1] + '/s | FRITZ!Box WAN Download | |' data += '| C | WAN Upload: ' + fc.str_transmission_rate[0] + '/s | FRITZ!Box WAN Upload | |' os.system('/usr/bin/qdbus org.kde.plasma.doityourselfbar /id_' + sys.argv[1] + ' org.kde.plasma.doityourselfbar.pass \'' + data + '\'') time.sleep(5)
Pretty neat. Thanks a lot to Klaus Bremer and the other fritzconnection authors and wsdfhjxc for making this possible!